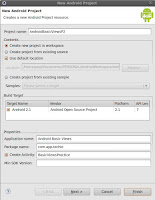
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<RadioGroup android:id="@+id/colorOptions"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
>
<RadioButton android:id="@+id/red"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Red"
/>
<RadioButton android:id="@+id/blue"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Blue"
/>
<RadioButton android:id="@+id/green"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Green"
/>
</RadioGroup>
</LinearLayout>
package com.app.techie;
import android.app.Activity;
import android.os.Bundle;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.Toast;
import android.widget.RadioGroup.OnCheckedChangeListener;
public class BasicViewsPractice extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.basicviewspart2);
RadioGroup colorChoices = (RadioGroup) findViewById(R.id.colorOptions);
colorChoices.setOnCheckedChangeListener(new OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
RadioButton redOption = (RadioButton) findViewById(R.id.red);
RadioButton blueOption = (RadioButton) findViewById(R.id.blue);
RadioButton greenOption = (RadioButton) findViewById(R.id.green);
if(redOption.getId()==checkedId){
displayToast("You selected red!");
}
if(blueOption.getId()==checkedId){
displayToast("You selected blue!");
}
if(greenOption.getId()==checkedId){
displayToast("You selected green!");
}
}
});
}
private void displayToast(String message){
Toast.makeText(getBaseContext(), message, Toast.LENGTH_SHORT).show();
}
}
<ProgressBar
android:id="@+id/normalProgressBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:visibility="gone"
/>
<Button android:id="@+id/btnDownload"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Download"
/>
package com.app.techie;
import android.app.Activity;
import android.os.Bundle;
import android.os.Handler;
import android.view.View;
import android.widget.Button;
import android.widget.ProgressBar;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.Toast;
import android.widget.RadioGroup.OnCheckedChangeListener;
public class BasicViewsPractice extends Activity {
private static int progress = 0;
private ProgressBar normalProgressBar;
private int progressStatus = 0;
private Handler handler = new Handler();
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.basicviewspart2);
RadioGroup colorChoices = (RadioGroup) findViewById(R.id.colorOptions);
colorChoices.setOnCheckedChangeListener(new OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
RadioButton redOption = (RadioButton) findViewById(R.id.red);
RadioButton blueOption = (RadioButton) findViewById(R.id.blue);
RadioButton greenOption = (RadioButton) findViewById(R.id.green);
if(redOption.getId()==checkedId){
displayToast("You selected red!");
}
if(blueOption.getId()==checkedId){
displayToast("You selected blue!");
}
if(greenOption.getId()==checkedId){
displayToast("You selected green!");
}
}
});
normalProgressBar = (ProgressBar) findViewById(R.id.normalProgressBar);
Button download = (Button) findViewById(R.id.btnDownload);
download.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//show the progress bar
normalProgressBar.setVisibility(0);
simulateProgress();
}
private void simulateProgress() {
progress = 0;
progressStatus = 0;
//do some work in background thread
new Thread(new Runnable()
{
public void run()
{
//do some work here
while (progressStatus < 10)
{
progressStatus = doSomeWork();
}
//hides the progress bar
handler.post(new Runnable()
{
public void run()
{
//0 - VISIBLE; 4 - INVISIBLE; 8 - GONE
normalProgressBar.setVisibility(8);
}
});
}
//do some long lasting work here
private int doSomeWork()
{
try {
//simulate doing some work
Thread.sleep(500);
} catch (InterruptedException e)
{
e.printStackTrace();
}
return ++progress;
}
}).start();
}
});
}
/**
* Helper method to display message
* @param message
*/
private void displayToast(String message){
Toast.makeText(getBaseContext(), message, Toast.LENGTH_SHORT).show();
}
}
<ProgressBar
android:id="@+id/normalProgressBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:visibility="gone"
style="?android:attr/progressBarStyleHorizontal"
/>
//do some work here
while (progressStatus < 100)
{
progressStatus = doSomeWork();
//Update the progress bar
handler.post(new Runnable()
{
public void run() {
normalProgressBar.setProgress(progressStatus);
}
});
}
What we are going to do first is update the android layout xml by adding an autocomplete textview:
<AutoCompleteTextView
android:id="@+id/txtFriends"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
final String[] friends = {
"Jose Rizal",
"Britney Spears",
"Sarah Walker",
"Sarah Jane",
"Brook How",
"Kimberly Some",
"Kimkrek Shoo"
};
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this,android.R.layout.simple_dropdown_item_1line, friends);
AutoCompleteTextView txtFriend = (AutoCompleteTextView) findViewById(R.id.txtFriends);
txtFriend.setThreshold(3);
txtFriend.setAdapter(adapter);
1 comments :
nice one gaw!
Post a Comment