Sunday, August 1, 2010
Software Engineer and Trainee
This tutorial is to create simple blogger template, with simple methods. The easiest way to create blogger template is by edit a template. But don't worry, althought this is a simple methods but it can produce a beautifull template, it depend on how you design it. This tutorial is not to create blogger template from beginning but this is to create template by editing a template. Ok lets begin.
Before we begin I suggest you to create a new blog,weI don't wont to destroy your blog.
To follow this tutorial you have to download this template first. downlad template.
We will create a template that contain four main elements, (Bakground, Header, Main and Footer) Like the image :
Before we begin I suggest you to create a new blog,weI don't wont to destroy your blog.
To follow this tutorial you have to download this template first. downlad template.
We will create a template that contain four main elements, (Bakground, Header, Main and Footer) Like the image :
Saturday, May 29, 2010
Combine Jetty and Spring Application Context XML Configuration
The goal is to use one xml configuration file which is Spring's applicationContext.xml and load it only once for the duration of the application. Using Embedded jetty as my lightweight server to run my Spring MVC application, I must tell my dispatcher servlets to use spring applicationContext.xml which already been loaded as its parent context. That way, dispatcher servlet will be able to referenced bean configured on springs application context.
To do that, create a class that extends org.mortbay.jetty.Server and implement org.springframework.context.ApplicationContextAware. This is pretty straight forward, we extend jetty server so we can configure its parent context. We implemented spring application context aware so whenever spring successfully loaded applicatContext.xml bean configuration, it gets the current application context. We then configure it as parent context of servlets.
Code on ServerConfigurer.java:
package com.sample.config;
import org.mortbay.jetty.Server;
import org.mortbay.jetty.servlet.ServletHandler;
import org.mortbay.jetty.webapp.WebAppContext;
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.web.context.WebApplicationContext;
import org.springframework.web.context.support.GenericWebApplicationContext;
public class ServerConfigurer
extends Server
implements ApplicationContextAware
{
private String _webAppDir = null;
private String _contextPath = null;
private ServletHandler _servletHandler = null;
private static ApplicationContext _applicationContext = null;
public String getContextPath() {
return _contextPath;
}
public ServletHandler getServletHandler() {
return _servletHandler;
}
public String getWebAppDir() {
return _webAppDir;
}
/**
* @see org.springframework.context.ApplicationContextAware#setApplicationContext(org.springframework.context.ApplicationContext)
*/
@Override
public void setApplicationContext(ApplicationContext applicationContext)
throws BeansException
{
_applicationContext = applicationContext;
}
public void setContextPath(String contextPath) {
_contextPath = contextPath;
}
public void setServletHandler(ServletHandler servletHandler) {
_servletHandler = servletHandler;
}
public void setWebAppDir(String webAppDir) {
_webAppDir = webAppDir;
}
@Override
protected void doStart()
throws Exception
{
final WebAppContext webAppContext = new WebAppContext(getServer(), _webAppDir, _contextPath);
final GenericWebApplicationContext webApplicationContext = new GenericWebApplicationContext();
webApplicationContext.setServletContext(webAppContext.getServletContext());
webApplicationContext.setParent(_applicationContext);
webAppContext.getServletContext().setAttribute(WebApplicationContext.ROOT_WEB_APPLICATION_CONTEXT_ATTRIBUTE, webApplicationContext);
webApplicationContext.refresh();
webAppContext.setServletHandler(_servletHandler);
addHandler(webAppContext);
super.doStart();
}
}
Text on bold note: Those are the pieces of code that basically are the most important in the server configurer. The setApplicationContext() method is the one that configures that gets the application context loaded by spring. The method doStart() is the one that does the story telling to web app that a current context has been loaded and it is the context should be used.
Code on applicationContext.xml:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.0.xsd" >
<!-- more beans configured -->
<bean name="webServer" class="point to your server configurer java file" init-method="start">
<property name="connectors">
<list>
<bean class="org.mortbay.jetty.nio.SelectChannelConnector">
<property name="host" value="${jetty.host}"/>
<property name="port" value="${jetty.port}"/>
</bean>
</list>
</property>
<property name="webAppDir" value="${jetty.webApp.dir}"/>
<property name="contextPath" value="${jetty.context.Path}"/>
<property name="servletHandler">
<bean class="org.mortbay.jetty.servlet.ServletHandler">
<property name="servlets">
<list>
<bean class="org.mortbay.jetty.servlet.ServletHolder">
<property name="name" value="dispatcher" />
<property name="servlet">
<bean class="org.springframework.web.servlet.DispatcherServlet" />
</property>
</bean>
</list>
</property>
<property name="servletMappings">
<list>
<bean class="org.mortbay.jetty.servlet.ServletMapping">
<property name="servletName" value="dispatcher" />
<property name="pathSpec" value="*.htm" />
</bean>
</list>
</property>
</bean>
</property>
<property name="handlers">
<list>
<!-- log handler -->
<bean class="org.mortbay.jetty.handler.RequestLogHandler">
<property name="requestLog">
<bean class="org.mortbay.jetty.NCSARequestLog">
<property name="append" value="true"/>
<property name="filename" value="${http.log.dir}/access.log.yyyy_mm_dd"/>
<property name="extended" value="true"/>
<property name="retainDays" value="999"/>
<property name="filenameDateFormat" value="yyyy-MM-dd"/>
</bean>
</property>
</bean>
</list>
</property>
</bean>
</beans>
That xml file comprise server configuration of your jetty server pointing dispatcher servlet use Spring's application context. Your next step is run your application the way you want.
I also did another article related to Spring MVC/IOC-DI and Embedded Jetty utilizing applicationContext .xml of Spring. You might be interested running and configuring jetty through java code and not from applicationContext.xml.
example:
_applicationContext = new ClassPathXmlApplicationContext(_resourceLocations);
_applicationContext.start();
I also did another article related to Spring MVC/IOC-DI and Embedded Jetty utilizing applicationContext .xml of Spring. You might be interested running and configuring jetty through java code and not from applicationContext.xml.
Hope this helps! For suggestion, please feel free to leave your comments.
Use Arguments on Property File
The goal on this tutorial is to be able to format a value on a properties file given its parameter or arguments. It is best describe as passing value as a parameter and inserting those values on the string retrieved from the properties file.
To better understand what I'm doing, read and understand my example code below.
Property File Sample
message.welcome= Welcome {0} to {1}!
message.thank= Thank you for you visit {0}.
ResourceBundle resBundle = new ResourceBundle("path to property file");
String welcomeText=resBundle.getString("message.welcome");
MessageFormat msgFormatter = new MessageFormat(welcomeText);
// Create the dynamic args you want to replace
Object[] messageArguments = {"Techie boy","Philippines"};
String finalText= msgFormatter.format(messageArguments);
The finalText will result to "Welcome Techie boy to Philippines!"
Wednesday, May 26, 2010
Embedded Jetty in Spring MVC, IoC/DI
I was trying to configure embedded jetty in a spring application that implements MVC(Model View Controller). I want to utilize single spring applicationContext xml file for IoC(Inversion of Control) and DI(Dependency Injection) on dispatcher servlets.
The goal is to be able to use or reference beans configured/located on already been loaded spring application context. Read and understand my resolution below.
The goal is to be able to use or reference beans configured/located on already been loaded spring application context. Read and understand my resolution below.
This is what my main method look like:
LOG.info("Application starting");
_applicationContext = new ClassPathXmlApplicationContext(_resourceLocations);
_webServer = new Server();
SelectChannelConnector connector = new SelectChannelConnector();
connector.setHost("localhost");
connector.setPort(8080);
_webServer.addConnector(connector);
WebAppContext webAppContext = new WebAppContext(_webServer, "path to web app folder", "/");
GenericWebApplicationContext webApplicationContext = new GenericWebApplicationContext();
webApplicationContext.setServletContext(webAppContext.getServletContext());
webApplicationContext.setParent(_applicationContext);
webAppContext.getServletContext().setAttribute(WebApplicationContext.ROOT_WEB_APPLICATION_CONTEXT_ATTRIBUTE, webApplicationContext);
webApplicationContext.refresh();
ServletHandler servletHandler = new ServletHandler();
ServletHolder servletHolder = new ServletHolder(new DispatcherServlet());
servletHolder.setName("dispatcher");
servletHandler.addServlet(servletHolder);
ServletMapping servletMapping = new ServletMapping();
servletMapping.setServletName(servletHolder.getName());
servletMapping.setPathSpec("*.htm");
servletHandler.addServletMapping(servletMapping);
webAppContext.setServletHandler(servletHandler);
_webServer.addHandler(webAppContext);
RequestLogHandler logHandler = new RequestLogHandler();
NCSARequestLog ncsaLog = new NCSARequestLog();
ncsaLog.setExtended(true);
ncsaLog.setFilename("logs/jetty-yyyy_mm_dd.log");
logHandler.setRequestLog(ncsaLog);
_webServer.addHandler(logHandler);
LOG.info("Starting main application context");
_applicationContext.start();
LOG.info("Starting Jetty Server");
try {
_webServer.start();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
LOG.info("Application started");
return null;
The idea is that DispatcherServlet's context is child context of GenericWebApplicationContext which in turn is a child of ClassPathXmlApplicationContext. Explicitly invoking setParent() gives you the access to all beans from the web application context.
Hope this helps. Feel free to leave your comment or suggestions.
Saturday, May 22, 2010
SVN error on Eclipse: org.tigris.subversion.javahl.ClientException: Attempted to lock an already-locked dir
I met an svn eclipse error while attempting to committing to svn repository. I was thinking I might aswell share how I resolve this problem to everyone.
SVN error on Eclipse: org.tigris.subversion.javahl.ClientException: Attempted to lock an already-locked dir
org.tigris.subversion.javahl.ClientException: Attempted to lock an already-locked dir
svn: Working copy ‘D:\htdocs_svn\sites\all\modules’ locked
org.tigris.subversion.javahl.ClientException: Attempted to lock an already-locked dir
svn: Working copy ‘D:\htdocs_svn\sites\all\modules’ locked
To be able to get rid of it, I did the following:
1. Right click on project folder
2. Goto Team and click Cleanup
3. Try to commit again
In my understanding, it happens when there is a pending session which already locked the working directory copy. So, to get rid of it.. You'll need to remove the old session by deleting them through svn cleanup.
Good luck!
I'm still breathing
It's been a while since my last post! I had been very busy lately with my work... I miss writing articles. After weeks of hardcore work, I've learn a lot about spring and jetty and I would say, its worth working hard.
I have this task of setting up a project for development. Its a web application for testing. I've learned embedding jetty in a spring application implementing MVC(Model View Controller) design pattern. Other than that, it builds character. Patience really was important.
Anyway, not good sharing my story. Anyway, I can't wait to share my learnings. Later I'll write about those things I've learned..Just the basics..
Saturday, April 24, 2010
Asus PL30JT Technology Business Notebook
Asus showed a notebook of new businesses, PL30JT at CeBIT 2010, which comes with a stylish design. The new Asus notebook includes Turbo33 technology company claims to improve performance by 33%.

The PL30JT is fueled by Intel Core i5 processor and up to 4 GB of RAM. It is equipped with two graphics cards, the 1GB NVIDIA GeForce 310M dedicated graphics and integrated Intel GMA HD technology with Optimus. The professional notebook comes with a 13.3-inch 1366 × 768 LCD display and support for WiFi. It weighs only 1.7 kilograms, with an 8-cell battery. [via itechnews]
This post Asus PL30JT Technology Business Notebook brough to by Netbook Review. Visit Electronics Review for information about gadget and technology
Friday, April 23, 2010
Lenovo IdeaPad Y460 and Y560 Notebooks
Lenovo has not forgotten the home users usually at CES 2010, the company unveiled two new models, the Lenovo IdeaPad Y460 and Y560 notebooks, with options on the latest processors Core i3, i5 and i7 Intel. The IdeaPad Y460 has a 14-inch 1366 x 768, Core i3 or i5 processor, ATI Radeon HD5740/HD5650 and up to 320 GB hard drive, while the IdeaPad Y560 has a 15.6 screen inch Core i3, i5 and i7 CPU, ATI Radeon HD5730 graphics and up to 500 GB hard drive.

Both machines have up to 8 GB of RAM, Combo drive blu-ray/dvd and standard WiFi b / g / n. There are also optional Bluetooth, 3G and WiMAX. Include three USB 2.0 ports, a / eSATA combo USB, ExpressCard slot and HDMI, with Ethernet, audio in / out and a memory card reader multiformat. [via slashgear]
This post Lenovo IdeaPad Y460 and Y560 Notebooks brough to by Netbook Review. Visit Electronics Review for information about gadget and technology
Lenovo IdeaPad G460 and G560 Switchable Graphics Notebooks
The IdeaPad G460 has a 14-inch LED screen with backlight, while the G560 gets a screen of 15.6 inches. They have up to 500 GB of hard disk storage. They start at $ 699.99.

Lenovo also announced the V360 IdeaPad 13.3-inch IdeaPad V460 14-inch laptops SOHO market. They have Switchable Graphics and are powered by 2010 Intel Core processors. Both models starting at $ 749.99.
Lenovo is adding the new IdeaPad G460 and G560 notebook, along with Y560 and Y460. The new IdeaPad is improved by a new Intel Core processor with Intel Turbo Boost, HD integrated graphics and up to 8GB of DDR3 memory. [via itechnews]
This post Lenovo IdeaPad G460 and G560 Switchable Graphics Notebooks brough to by Netbook Review. Visit Electronics Review for information about gadget and technology
ASUS Eee PC 1005P Minimalist Netbook
Recently, detailed specifications and pictures of 1005P ASUS Eee PC showed on SlashGear, the netbook has a minimalist design with a pretty decent hardware spec.

This netbook features a 10.1-inch screen supports a resolution of 1024 × 600, it also features an Intel Atom N450 (1.66GHz) processor, 1 GB of RAM and Intel GMA 3150 graphics. You can choose between a 160 GB hard drive 250GB and the netbook has WiFi b / g standard, the more impressive as it offers up to 12 hours of battery life.
The Eee PC 1005P is currently available in the UK for £ 242.95, while the 250GB model (1005PE) is the sale of £ 270.34, these prices equivalent to approximately $ 395 and $ 439 respectively. [via product-reviews]
This post ASUS Eee PC 1005P Minimalist Netbook brough to by Netbook Review. Visit Electronics Review for information about gadget and technology
ASUS Eee PC 1001P Netbook
Plus in Germany, the ASUS Eee PC 1001P has been made available for pre-order costing € 249 which is the equivalent of about $ 357 USD.

The netbook runs Windows XP rather than Windows 7, which we would have expected. It also has a smaller capacity battery than other standard models that will probably limit their use to less than 10 hours. What it does come with a new Intel Atom N450 processor to be launched January 6, which is also the official launch of the Eee Netbook 1001P.
Other features of the netbook include wireless 802.11b / g speeds, 3 x USB 2.0 ports and a 0.3 megapixel webcam. Memory wise it has 1 GB hard disk has a capacity of 160GB. The screen measures 10.1 inches is expected throughout Netbook Eee 1000 series. [via itechnews]
This post ASUS Eee PC 1001P Netbook brough to by Netbook Review. Visit Electronics Review for information about gadget and technology
Dell Vostro 3000 Slim Lightweight Notebook
Following the V13 Vostro, Dell offers the new Vostro 3000 series, a range of new laptops thin, lightweight and robust design for small businesses. Spurred by the latest Intel Core Series, Vostro 3000 is up to 6 GB of memory.

New Dell Vostro 3000 series includes the 13.3-inch Vostro 3300 which is one of the thinnest commercial 13-inch laptop with integrated optical drive, the 14-inch Vostro 3400 with up to 8 hours of autonomy, and the 17 inch Vostro 3700 with an optional Core i7 quad core processors.
The Vostro 3500 and 3700 models come with LED backlight and it can be equipped with up to 1GB NVIDIA GeForce dedicated. The new Vostro laptops support Bluetooth, WiFi and mobile broadband WWAN optional. These new phones will be released March 16. [via itechnews]
This post Dell Vostro 3000 Slim Lightweight Notebook brough to by Netbook Review. Visit Electronics Review for information about gadget and technology
Tuesday, April 20, 2010
Add Related Posts Widget to Blogger or Blogspot
It be better to display related or similar articles below your articles or posts on your blog. This way your readers would easily know that there are related articles you written and they can further read.
The steps to adding related posts widget is easy. It would take less than 3 minutes add it on your blog.
Before starting, make sure to download the related post JavaScript file or ccopy and paste its content to your desired file.js. Then make sure to upload it online where it will be available for import on your template. I recommend you to upload it on Weebly.com, SigMirror.com or HotLinkFiles.com to get a DIRECT LINK to that file.
Step 1: Most important of all, backup your template incase something go wrong or messed up. Goto www.blogspot.com "Layout" portion, then go to "Edit HTML" section. Download present template and make sure to secure the copy on your file storage device.
Step 2: Click on "Expand widget templates" and look for this code:
</head>
and on top of it insert the following code:
<!--RelatedPostsStarts-->
<style>
#related-posts {
float : left;
width : 540px;
margin-top:20px;
margin-left : 5px;
margin-bottom:20px;
font : 11px Verdana;
margin-bottom:10px;
}
#related-posts .widget {
list-style-type : none;
margin : 5px 0 5px 0;
padding : 0;
}
#related-posts .widget h2, #related-posts h2 {
color : #940f04;
font-size : 20px;
font-weight : normal;
margin : 5px 7px 0;
padding : 0 0 5px;
}
#related-posts a {
color : #054474;
font-size : 11px;
text-decoration : none;
}
#related-posts a:hover {
color : #054474;
text-decoration : none;
}
#related-posts ul {
border : medium none;
margin : 10px;
padding : 0;
}
#related-posts ul li {
display : block;
margin : 0;
padding-top : 0;
padding-right : 0;
padding-bottom : 1px;
padding-left : 16px;
margin-bottom : 5px;
line-height : 2em;
border-bottom:1px dotted #cccccc;
}
</style>
<script src='http://www.weebly.com/..../relatedposts_forblogger.js' type='text/javascript'/>
<!--RelatedPostsStops-->
Replace the red link above with your own direct link.
Step 3: Look for the code:
<data:post.body/>
Under it, insert the following code:
<!--RELATED-POSTS-STARTS--><b:if cond='data:blog.pageType == "item"'>
<div id='related-posts'>
<font face='Arial' size='3'><b>Related Posts: </b></font><font color='#FFFFFF'><b:loop values='data:post.labels' var='label'><data:label.name/><b:if cond='data:label.isLast != "true"'>,</b:if><b:if cond='data:blog.pageType == "item"'>
<script expr:src='"/feeds/posts/default/-/" + data:label.name + "?alt=json-in-script&callback=related_results_labels&max-results=5"' type='text/javascript'/></b:if></b:loop> </font>
<script type='text/javascript'> removeRelatedDuplicates(); printRelatedLabels();
</script></div></b:if><!--RELATED-POSTS-STOPS-->
To limit the maximum number of related or similar articles to display under the post, just change max-results=xx by the number you like.
Finally, save your template and test. Related Posts widget should be located under your article or post. Do remember to add categories or labels before you publish your post. Otherwise, no related post will be displayed.
Hope this tutorial helps.
Friday, April 16, 2010
Tutorial on Android Views - Part 2
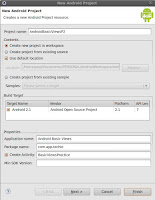
Lets start by creating a new android project on eclipse. See the screenshot beside for android project specifics.
RadioGroup and RadioButton
This two views is basically used to let users choose something among available options. RadioButton has two available states. It is either checked or unchecked. Once a RadioButton is checked, it cannot be unchecked unless its grouped along with other radio button on a RadioGroup. A RadioGroup is a type of view that is used to group one or mmore radio buttons, thereby allows a user to select only one radiobutton or only one option.
Create new android xml named basicviewspart2.xml under res/layout folder then put the following code:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<RadioGroup android:id="@+id/colorOptions"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
>
<RadioButton android:id="@+id/red"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Red"
/>
<RadioButton android:id="@+id/blue"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Blue"
/>
<RadioButton android:id="@+id/green"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Green"
/>
</RadioGroup>
</LinearLayout>
Then, update BasicViewsPractice.java by adding the following codes:
package com.app.techie;
import android.app.Activity;
import android.os.Bundle;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.Toast;
import android.widget.RadioGroup.OnCheckedChangeListener;
public class BasicViewsPractice extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.basicviewspart2);
RadioGroup colorChoices = (RadioGroup) findViewById(R.id.colorOptions);
colorChoices.setOnCheckedChangeListener(new OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
RadioButton redOption = (RadioButton) findViewById(R.id.red);
RadioButton blueOption = (RadioButton) findViewById(R.id.blue);
RadioButton greenOption = (RadioButton) findViewById(R.id.green);
if(redOption.getId()==checkedId){
displayToast("You selected red!");
}
if(blueOption.getId()==checkedId){
displayToast("You selected blue!");
}
if(greenOption.getId()==checkedId){
displayToast("You selected green!");
}
}
});
}
private void displayToast(String message){
Toast.makeText(getBaseContext(), message, Toast.LENGTH_SHORT).show();
}
}
Briefly, the code above simply display a message through Toast. the message indicates what option a user selects. We identified the radiobutton selected using its IDs. The above should look like:
ProgressBar
This view is usually used to indicate that an event, task or an activity is currently ongoing. An example of which is downloading files where progress of download is shown visually though a color bar. This is a good choice to tell the user about the status of the task. For us to understand it better, lets do the activity below.
Lets use same activity created above and update the basicviewspart2.xml by adding the following code:
<ProgressBar
android:id="@+id/normalProgressBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:visibility="gone"
/>
<Button android:id="@+id/btnDownload"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Download"
/>
On our xml code, we added two views. A progress bar with visibility default to gone so at startup it does not appear on display and a button to do some work or task on background. What we want to do is to simulate progress bar. So, if the user clicks the button, a task is started and the progress bar will display and hide when the task is done.
Update BasicActivityPractice.java to look like this:
package com.app.techie;
import android.app.Activity;
import android.os.Bundle;
import android.os.Handler;
import android.view.View;
import android.widget.Button;
import android.widget.ProgressBar;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.Toast;
import android.widget.RadioGroup.OnCheckedChangeListener;
public class BasicViewsPractice extends Activity {
private static int progress = 0;
private ProgressBar normalProgressBar;
private int progressStatus = 0;
private Handler handler = new Handler();
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.basicviewspart2);
RadioGroup colorChoices = (RadioGroup) findViewById(R.id.colorOptions);
colorChoices.setOnCheckedChangeListener(new OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
RadioButton redOption = (RadioButton) findViewById(R.id.red);
RadioButton blueOption = (RadioButton) findViewById(R.id.blue);
RadioButton greenOption = (RadioButton) findViewById(R.id.green);
if(redOption.getId()==checkedId){
displayToast("You selected red!");
}
if(blueOption.getId()==checkedId){
displayToast("You selected blue!");
}
if(greenOption.getId()==checkedId){
displayToast("You selected green!");
}
}
});
normalProgressBar = (ProgressBar) findViewById(R.id.normalProgressBar);
Button download = (Button) findViewById(R.id.btnDownload);
download.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//show the progress bar
normalProgressBar.setVisibility(0);
simulateProgress();
}
private void simulateProgress() {
progress = 0;
progressStatus = 0;
//do some work in background thread
new Thread(new Runnable()
{
public void run()
{
//do some work here
while (progressStatus < 10)
{
progressStatus = doSomeWork();
}
//hides the progress bar
handler.post(new Runnable()
{
public void run()
{
//0 - VISIBLE; 4 - INVISIBLE; 8 - GONE
normalProgressBar.setVisibility(8);
}
});
}
//do some long lasting work here
private int doSomeWork()
{
try {
//simulate doing some work
Thread.sleep(500);
} catch (InterruptedException e)
{
e.printStackTrace();
}
return ++progress;
}
}).start();
}
});
}
/**
* Helper method to display message
* @param message
*/
private void displayToast(String message){
Toast.makeText(getBaseContext(), message, Toast.LENGTH_SHORT).show();
}
}
The above code retrieves the progress bar we added to layout earlier as well as the button. When the button is clicked, the progress bar visibility is changed to visible mode and a background task simulation took place wherein upon its done, the visibility of the progress bar is changed back to gone. By the way, there are three representations of visibility - 0 for visible, 4 for invisible, and 8 for gone. The activity should look like below.
Moreover, ProgressBar has an attribute style wherein you can change its display. Instead of intermediate mode style like above, you can use a horizontal bar. Take note of the code:
<ProgressBar
android:id="@+id/normalProgressBar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:visibility="gone"
style="?android:attr/progressBarStyleHorizontal"
/>
And then change your activity class code in method simulationProgress() to:
//do some work here
while (progressStatus < 100)
{
progressStatus = doSomeWork();
//Update the progress bar
handler.post(new Runnable()
{
public void run() {
normalProgressBar.setProgress(progressStatus);
}
});
}
The result should be like:
AutoCompleteTextView
It is a subclass of textview only that it has an auto complete suggestion feature. Think of a textbox while typing and under it is keyword suggestions. This basically helps you reduce the typing effort and typo error.
What we are going to do first is update the android layout xml by adding an autocomplete textview:
<AutoCompleteTextView
android:id="@+id/txtFriends"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
Afterwards, we add code to our activity class inside onCreate method:
final String[] friends = {
"Jose Rizal",
"Britney Spears",
"Sarah Walker",
"Sarah Jane",
"Brook How",
"Kimberly Some",
"Kimkrek Shoo"
};
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this,android.R.layout.simple_dropdown_item_1line, friends);
AutoCompleteTextView txtFriend = (AutoCompleteTextView) findViewById(R.id.txtFriends);
txtFriend.setThreshold(3);
txtFriend.setAdapter(adapter);
Understanding the code above: We made an array of string where its value will be the value suggested to the user. It is feed into an ArrayAdapter object where the auto complete textview can obtain suggestions. If you notice, we also set threshold to 3. Threshold is used to set the minimum characters before suggestions appear as a drop-down menu. Try running the application and it should look like:
Nutshell
Finally, we are donw with those another three kind of useful Android views. In this tutorial we've learned more basic on views. Another article is coming up taking up more views so stay tuned. Hope this article helps you.
Thursday, April 15, 2010
Check If a String Contains a Substring in Java
This article shows some useful ways on checking a string that contains a substring. I find this topic relevant to document as I may someday use this on another project. Also, other developers might look for an article online to help them solve their problem. Since this topic is very basic Java, I only added short description and sample code below.
//Create a string to evaluate
String phrase = "The big brown fox jumped over the lazy dog!";
//Check if it contains a case sensitive substring
boolean evaluation = phrase.contains("brown"); //returns true
evaluation = phrase.contains("Brown"); //returns false
evaluation = phrase.indexOf("fox jumped") > 0; //returns true
//Check if phrase starts with a substring
evaluation = phrase.startsWith("Th"); //returns true
//Check if phrase ends with a substring
evaluation = phrase.endsWith("og"); //returns true
//To check string with ignore case, we should be using regular expression
evaluation = string.matches("(?i).*i am.*"); //returns true
//Check if phrase starts with a substring
evaluation = string.matches("(?i)th.*"); //returns true
//Check if phrase ends with a substring
evaluation = string.matches("(?i).*adam"); //returns true
That is all for this article. I hope this helps you.
Wednesday, April 14, 2010
Best Tool for Monitoring Social Media or Websites
I found a great site or tool for monitoring social media or social networking websites like Twitter, Facebook, FriendFeed, YouTube, Digg, Google etc. If you wanted to know what people are saying about you, an event, any person or anything, I recommend you do a visit and perhaps, try out Social Mention services.
They have a Realtime Buzz Widget which is very interesting, very useful and very easy to use or integrate on any blog platform whether it be Wordpress, Blogger or others. It is as easy as adding a JavaScript widget into your blog template. It does not require programming skills. all you need to do is to copy the html, set the search phrase and title, and put it on your site. The code below are the only code you'll need to add into your blog.
//See latest code on http://socialmention.com/tools/ page.
//***********************************************************
<script type="text/javascript">
// search phrase (replace this)
var smSearchPhrase = 'socialmention';
// title (optional)
var smTitle = 'Realtime Buzz';
// items per page
var smItemsPerPage = 7;
// show or hide user profile images
var smShowUserImages = true;
// widget font size in pixels
var smFontSize = 11;
// height of the widget
var smWidgetHeight = 500;
// sources (optional, comment out for "all")
//var smSources = ['twitter', 'googleblog', 'brightkite', 'delicious', 'friendfeed', 'flickr', 'identica', 'youare', 'digg'];
</script>
<script type="text/javascript" language="javascript" src="http://socialmention.com/widgets/buzz.js"></script>
The code will result to:
In my opinion and as a person who likes to write, read and share articles online and loves the internet, I find this widget very helpful and useful. Thanks to Jon Cianciullo ,the person behind this project that allows us to easily track and measure what people are saying about you, your company, a new product, or any topic across the web's social media landscape in real-time.
Saturday, April 10, 2010
Android Software Competition by Globe Telecom
I first heard this news from Calen Legaspi, our CEO at Orange and Bronze Software Labs, that Globe Labs will host and conduct its first mobile developer event for 2010. I've been hearing about Android platform since 2008 and now, Globe Labs posted an invitation for all developers to explore the new mobile platform.
The event is an Android software competition open for all Filipino developers. This is an invitation to all developers to build their mobile application on top of Android platform.
Big cash prizes are at stake in this competition, as well as the chance for the participating teams to pitch their product to international investors for funding. The competition will run through until July 2010. For more details on contest mechanics, dates and guidelines, please click here.
I think this event is a great opportunity for all developers. Its not just an opportunity to make money, but, an opportunity for learning how to develop an application on a new mobile platform.
To those who are interested to join this competition, visit Globe Labs website at www.globelabs.com to sign-up.
Tutorial on Android Views - Part 1
On my previous articles, we had a discussion on the android's definition and layout. I assume that you have already learn basics on manipulating android layout. In this and on the next article, we will be exploring and dealing more on the various types of commonly used views such as TextView, EditText, Button, ImageButton, CheckBox and ToggleButton. Those widgets are likely the most commonly used in developing android applications.
Lets begin by creating a new project on eclipse:
After which, create an Android XML under res/layout and name it basicviews.xml having written inside is the following code below:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
>
<TextView
android:text="Welcome to Android!"
android:layout_height="wrap_content"
android:layout_width="wrap_content"
/>
<EditText
android:id="@+id/sampleText"
android:text=""
android:layout_height="wrap_content"
android:layout_width="fill_parent"
/>
<Button
android:id="@+id/popupSampleText"
android:text="Pop up"
android:layout_height="wrap_content"
android:layout_width="fill_parent"
/>
</LinearLayout>
The code above contains basic views such as textview, edittext and button supplied with an id. The views id will be used later and discussed further in this discussion. We are doing this layout so we will be able to play around with those views. Below is a little description on those view types.
- TextView - is basically an element that is used to display text on screen or to the user. It is one of the most basic views and most commonly used in developing an android application. Also, do take note that the text inside this view type is not editable.
- EditText - is another type of view that is used for character/text input such as name or passwords.
- Button - it represents a push-button widget which can be pushed or clicked by the user to perform an activity, an event or an action.
package com.app.techie;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
public class BasicViewsPractice extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.basicviews);
Button popUp = (Button) findViewById(R.id.popupSampleText);
popUp.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
TextView sampleText = (TextView) findViewById(R.id.sampleText);
if(0 != sampleText.getText().toString().length()){
Toast.makeText(getBaseContext(), sampleText.getText().toString(), Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(getBaseContext(), "The textbox is empty!", Toast.LENGTH_SHORT).show();
}
}
});
}
}
In the code above, instead of using the main.xml layout, we replace it with basicviews.xml layout on setContentView(...) method to load the layout we just did a while ago. We also instantiated a button as well as a editview. Those views are linked to the corresponding element with the exact id on the xml layout. Moreover, we also added an onClickListener () to the button so whenever the user clicks on it, the text inside the edittext will display on screen through Toast. Try running the application and it should look like this:
There you go! Your application should be similar to above. If the edittext is empty, it should display "textbox is empty" as a message. Moving forward, add the code below on basicviews.xml just right after popupButton view.
<EditText
android:id="@+id/sampleTextPassword"
android:text="sample"
android:password="true"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
/>
<ImageButton
android:id="@+id/sampleImageButton"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:src="@drawable/icon"
/>
The code added two new views. The edittext character will be replaced with a dot since we set its password attribute to true. The imagebutton is similar to a normal button view. Only that image button has an attribute where you can specify an icon to display wrap inside a button.
Lets do another update by adding the following code:
<CheckBox android:id="@+id/chkSample"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Checkbox Sample"
/>
<CheckBox android:id="@+id/chkStarLook"
style="?android:attr/starStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Star checkbox"
/>
On the code, the first checkbox will look normal with a square and the second one will appear like a star since we provided a starStyle to its attribute. A checkbox is another special type of button. It has two state and its default is unchecked, otherwise its checked. For us to be able to try out checkbox view state, lets update BasicViewsPractice.java by adding the following code below:
CheckBox chkSample = (CheckBox) findViewById(R.id.chkSample);
chkSample.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if(((CheckBox) v).isChecked()){
Toast.makeText(getBaseContext(), "Checked", Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(getBaseContext(), "Unchecked", Toast.LENGTH_SHORT).show();
}
}
});
That suffice how you are going to verify a chechbox view state using java code. That same code also applies to the star checkbox. Just change the id of the checkbox on your java code. Try running the code and you should be able to see something like below.
Another view that behaves like CheckBox is the ToggleButton. It has two state, checked and unchecked, just like a CheckBox view. Only that this time, it has a light indicator to display its current state. Try it out by adding a togglebutton on basicviews.xml.
<ToggleButton android:id="@+id/tglSample"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Light switch"
/>
To be able to check its current state, add the following code below on the BasicViewsPractice.java.
ToggleButton tglSample = (ToggleButton) findViewById(R.id.tglSample);
tglSample.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if(((ToggleButton) v).isChecked()){
Toast.makeText(getBaseContext(), "Light On", Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(getBaseContext(), "Light Off", Toast.LENGTH_SHORT).show();
}
}
});
As you can see above, its more or less like the code on checking a checkbox view. Try running the code and see for yourself.
Nutshell:
In this tutorial we were able to learn and experiment on textView, edittext, button, imagebutton, checkbox and toggle button. However, there are still more views to learn and they will be discussed in detail my next article. Hope you learned from this article. Enjoy!
Subscribe to:
Posts
(
Atom
)